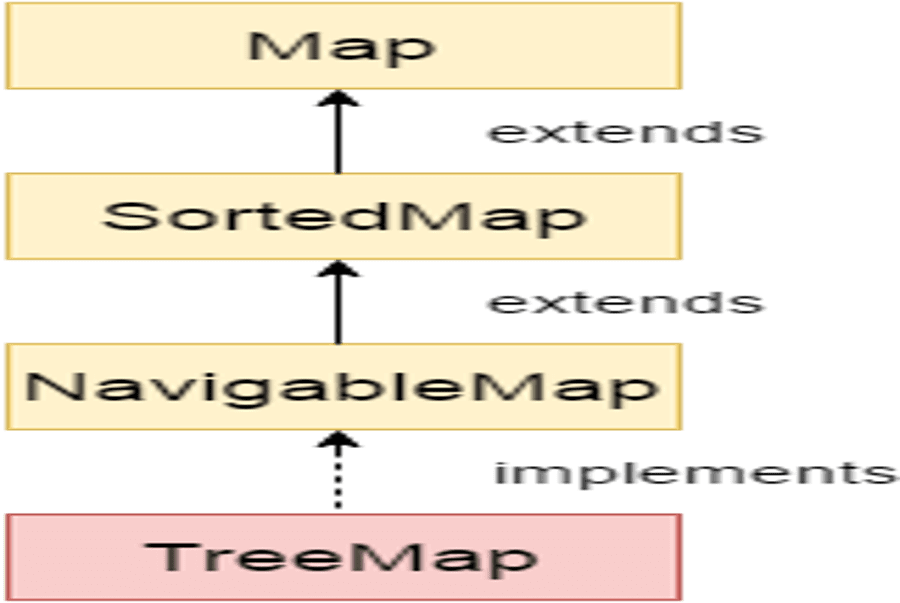
Tóm Tắt
Đặc điểm
Những điểm quan trọng về lớp TreeMap trong java cần nhớ là:
- TreeMap lưu trữ dữ liệu dưới dạng cặp key và value.
- TreeMap chỉ chứa các key duy nhất.
- TreeMap KHÔNG cho phép bất kỳ key nào là null và nhưng có thể có nhiều giá trị null.
- TreeMap duy trì các phần tử được thêm vào theo thứ tự key tăng dần.
Hierarchy của lớp TreeMap
Lớp java.util.TreeMap được định nghĩa như sau:
public class TreeMapextends AbstractMap implements NavigableMap , Cloneable, java.io.Serializable { }
Trong đó:
Bạn đang đọc: Lớp TreeMap trong Java – GP Coder (Lập trình Java)
- K: đây là kiểu key để lưu trữ.
- V: đây là kiểu giá trị được ánh xạ.
Các phương pháp khởi tạo ( constructor ) của lớp TreeMap
- LinkedHashMap(): khởi tạo một map trống.
- LinkedHashMap(Map extends K, ? extends V> m): khởi tạo một map với các phần tử của map m.
Các phương pháp ( method ) của lớp TreeMap
Xem thêm những phương pháp của Map ở bài viết Map Interface trong java .
Ví dụ minh họa
Ví dụ sử dụng TreeMap
với kiểu dữ liệu cơ bản (Wrapper)
package com.gpcoder.collection.treemap; import java.util.Map; import java.util.Map.Entry; import java.util.TreeMap; public class LinkedHashMapExample { public static void main(String args[]) { // init map Mapmap = new TreeMap (); map.put(1, "Basic java"); map.put(2, "OOP"); map.put(4, "Multi-Thread"); map.put(3, "Collection"); // show map using method keySet() for (Integer key : map.keySet()) { String value = map.get(key); System.out.println(key + " = " + value); } System.out.println("---"); // show map using method keySet() for (Entry entry : map.entrySet()) { Integer key = entry.getKey(); String value = entry.getValue(); System.out.println(key + " = " + value); } } }
Kết quả thực thi chương trình trên :
1 = Basic java 2 = OOP 3 = Collection 4 = Multi-Thread --- 1 = Basic java 2 = OOP 3 = Collection 4 = Multi-Thread
Ví dụ sử dụng TreeMap với key có kiểu String, value có kiểu Student
package com.gpcoder.collection.map; public class Student { private int id; private String name; public Student(int id, String name) { this.id = id; this.name = name; } @Override public String toString() { return "Student [id=" + id + ", name=" + name + "]"; } public int getId() { return id; } public String getName() { return name; } }
package com.gpcoder.collection.treemap; import java.util.Map; import java.util.Map.Entry; import java.util.TreeMap; public class LinkedHashMapExample2 { public static void main(String args[]) { // Student's data Student student1 = new Student(1, "Student 1"); Student student2 = new Student(2, "Student 2"); Student student3 = new Student(3, "Student 3"); Student student4 = new Student(4, "Student 4"); // init map Mapmap = new TreeMap (); map.put(student1.getId(), student1); map.put(student2.getId(), student2); map.put(student4.getId(), student4); map.put(student3.getId(), student3); // show map using method keySet() for (Integer key : map.keySet()) { Student value = map.get(key); System.out.println(key + " = " + value); } System.out.println("---"); // show map using method keySet() for (Entry entry : map.entrySet()) { Integer key = entry.getKey(); Student value = entry.getValue(); System.out.println(key + " = " + value); } } }
Kết quả thực thi chương trình trên :
1 = Student [id=1, name=Student 1] 2 = Student [id=2, name=Student 2] 3 = Student [id=3, name=Student 3] 4 = Student [id=4, name=Student 4] --- 1 = Student [id=1, name=Student 1] 2 = Student [id=2, name=Student 2] 3 = Student [id=3, name=Student 3] 4 = Student [id=4, name=Student 4]
4.8
Xem thêm: Constructor trong JavaScript
Nếu bạn thấy hay thì hãy chia sẻ bài viết cho mọi người nhé!
Shares
Bình luận
phản hồi
Source: https://final-blade.com
Category: Kiến thức Internet