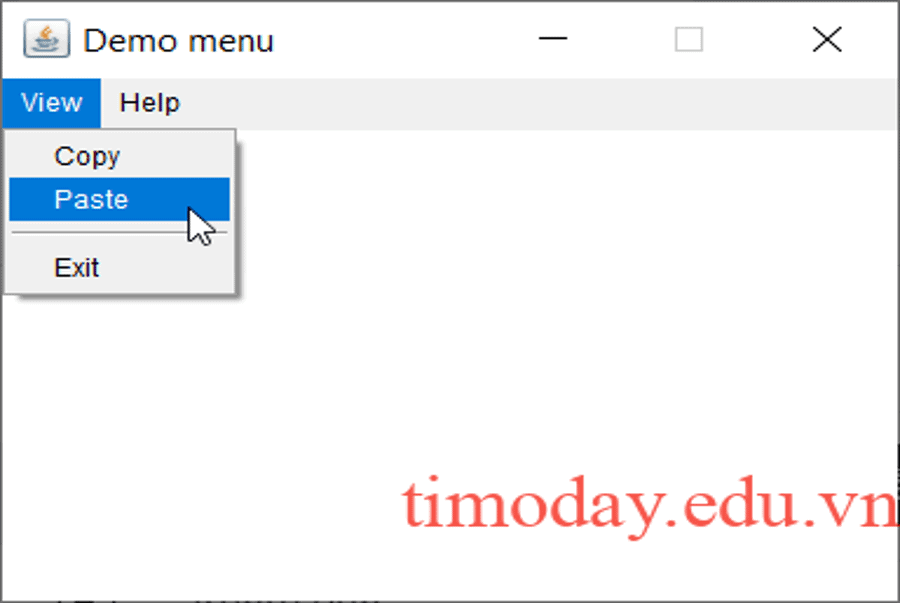
Đề bài: Viết chương trình giao diện gồm có hệ thống menu như sau: Thanh menu chính chứa 2 mục View và Help. Mục View chứa 3 mục con Copy, Paste và Exit, giữa mục Paste và Exit có một thanh ngang để ngăn cách. Mục Help chứa 2 mục con là Help view và About. Khi người dùng chọn mục Exit chương trình kết thúc và và chọn mục About chương trình sẽ hiển thị một số thông tin do người lập trình thiết lập.
Yêu cầu kiến thức:
- Kế thừa và triển khai các phương thức đã được đề ra ở các lớp cha
- Phân tích và thiết kế các đối tượng trên giao diện là các components
- Hiểu rõ bản chất cách hoạt động các đối tượng
Cấu trúc thư mục:
src
|——buildUI
|——DemoMenu.java
|——About.java
|——usingUI
|——MainClass.java
Code tham khảo dưới đây được viết trên JDK ver 8.x:
File DemoMenu.java:
package buildUI;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
public class DemoMenu extends Frame implements ActionListener {
// Tao menubar
private MenuBar menuBar;
// 2 menu chinh
private Menu view, help;
// Cac muc con cua cac menu chinh
private MenuItem exit, copy, paste, viewHelp, about;
// Ham khoi tao
public DemoMenu(String title) {
super(title);
// Tao thanh menu
menuBar = new MenuBar();
// Dat thanh menu vao giao dien
setMenuBar(menuBar);
// Tao va dat menu chinh View vao thanh menu
view = new Menu("View");
menuBar.add(view);
view.addActionListener(this);
// Tao cac menu con vao menu chinh View
copy = new MenuItem("Copy");
view.add(copy);
copy.addActionListener(this);
paste = new MenuItem("Paste");
view.add(paste);
paste.addActionListener(this);
// Ngan cach
view.addSeparator();
exit = new MenuItem("Exit");
view.add(exit);
exit.addActionListener(this);
// Tao menu chinh Help va dat vao thanh menu
help = new Menu("Help");
menuBar.add(help);
help.addActionListener(this);
// Tao cac menu con va dat vao menu chinh Help
viewHelp = new MenuItem("Help view");
help.add(viewHelp);
viewHelp.addActionListener(this);
about = new MenuItem("About");
help.add(about);
about.addActionListener(this);
// Thiet lap kich thuoc va hien thi Frame
setSize(400, 300);
setVisible(true);
// Khong cho phep thay doi kich thuoc giao dien
setResizable(false);
// Cho phep dung chuot de tat cua so
this.addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getActionCommand().equals("Exit")) {
System.exit(0);
}
if (e.getActionCommand().equals("About")) {
new About().setVisible(true);
}
}
}
File About.java:
Bạn đang đọc: Tạo và sử dụng Menu bar trong Java Swing | Tìm ở đây
package buildUI;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class About extends Frame implements ActionListener {
// Thuoc tinh
private Button btnClose;
private Label lbText1, lbText2, lbText3, lbText4;
// Ham khoi tao
public About() {
super("About");
setLayout(new FlowLayout(FlowLayout.CENTER));
btnClose = new Button("Close");
btnClose.setFont(new Font("Arial", Font.PLAIN, 18));
btnClose.setForeground(Color.lightGray);
btnClose.addActionListener(this);
lbText1 = new Label("Demo Menu version x");
lbText1.setFont(new Font("Arial", Font.PLAIN, 12));
lbText2 = new Label("Author: hvtuan");
lbText2.setFont(new Font("Arial", Font.PLAIN, 12));
lbText3 = new Label("email: [email protected]");
lbText3.setFont(new Font("Arial", Font.PLAIN, 12));
lbText4 = new Label("Tel: 1234567890");
lbText4.setFont(new Font("Arial", Font.PLAIN, 12));
add(lbText1);
add(lbText2);
add(lbText3);
add(lbText4);
add(btnClose);
setSize(200, 190);
setResizable(false);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == btnClose) {
this.setVisible(false);
}
}
}
File MainClass.java:
package usingUI;
import buildUI.DemoMenu;
public class MainClass {
public static void main(String[] args) {
DemoMenu demoMenu = new DemoMenu("Demo menu");
}
}
Kết quả chương trình:
Kết luận:
- Bạn có thể tham khảo thêm khóa học lập trình Java từ cơ bản đến nâng cao. Xem tại đây
- Bạn có thể tham khảo thêm khóa học lập trình C từ cơ bản đến nâng cao. Xem tại đây
- Bạn có thể tham khảo thêm khóa học Thành thạo lập trình C#. Xem tại đây
- Bạn có thể tham khảo thêm khóa học Ôn tập OOP cơ bản trong Java. Xem tại đây
Source: https://final-blade.com
Category: Kiến thức Internet